kqcircuits.elements.waveguide_composite
- class kqcircuits.elements.waveguide_composite.Node(position, element=None, inst_name=None, align=(), angle=None, length_before=None, length_increment=None, **params)[source]
Bases:
object
Specifies a single node of a composite waveguide.
Node is as a
position
and optionally other parameters. Theelement
argument sets an Element type that gets inserted in the waveguide. Typically this is an Airbridge, but any element with port_a and port_b is supported.- Parameters:
position – The location of the Node. Represented as a DPoint object.
element – The Element type that gets inserted in the waveguide. None by default.
inst_name – If an instance name is supplied, the element refpoints will be exposed with that name. Default None.
align – Tuple with two refpoint names that correspond the input and output point of element, respectively Default value (None) uses
('port_a', 'port_b')
angle – Angle of waveguide direction in degrees
length_before – Length of the waveguide segment before this node
length_increment – Waveguide length increment produced by meander before this node
**params – Other optional parameters for the inserted element
- Returns:
A Node.
- position: DPoint
- align: Tuple
- inst_name: str
- angle: float
- length_before: float
- length_increment: float
- classmethod deserialize(node)[source]
Create a Node object from a serialized form, such that
from_serialized(ast.literal_eval(str(node_object)))
returns an equivalent copy ofnode_obj
.- Parameters:
node – serialized node, consisting of a tuple
(x, y, element_name, params)
, wherex
andy
are the node coordinates. The stringelement_name
and dictparams
are optional.
Returns: a Node
- static nodes_from_string(nodes)[source]
Converts the human readable text representation of Nodes to an actual Node object list.
Needed for storage in KLayout parameters. The string has to conform to a specific format: (x, y, class_str, parameter_dict). For example (0, 500, ‘Airbridge’, {‘n_bridges’: 2}), see also the Node.__str__ method. Empty class_str or parameter_dict may be omitted.
- Returns:
list of Node objects
- class kqcircuits.elements.waveguide_composite.WaveguideComposite[source]
Bases:
Element
A composite waveguide made of waveguides and other elements.
From the user’s perspective this is a WaveguideCoplanar that is extended with Airbridge, WaveguideCoplanarTaper and FlipChipConnector, so that a signal can be routed over other waveguides and several chip faces. As a bonus feature other arbitrary elements like coplanar capacitors are also supported.
A list of Nodes defines the shape of the waveguide. An empty node, i.e.
Node.element
isNone
, will set the next “waypoint” of the waveguide. If an Element is given it will be placed on the node, except for the first or last node where it will be adjacent to the edge.Inserted elements are oriented collinear with the previous node. If the following node is not collinear with the previous element then for convenience an automatic bend is inserted in the waveguide. Note that back-to-back elements (i.e. without some empty Nodes in between) should only be placed collinear with each other.
Node parameters will be passed to the element. For convenience, empty nodes may also have parameters:
a
,b
orface_id
. They insert a WaveguideCoplanarTaper or a FlipChipConnector, respectively and change the defaults too.The
ab_across=True
parameter places a single airbridge across the node. Then_bridges=N
parameter puts N airbridges evenly distributed across the preceding edge. Ifn_bridges=-1
the number of bridges will be calculated automatically fromab_to_ab_spacing
andab_to_node_clearance
, with the former defining the density of airbridges and the latter the clearance from the start and end node of the straight segment.The
length_before
parameter of a node can be specified to automatically set the length of the waveguide between that node and the previous one. It will in fact create a Meander element instead of a normal waveguide between those nodes to achieve the correct length. Alternative parameterlength_increment
sets the waveguide length increment compared to normal waveguide.If
add_metal
parameter is set toTrue
for a node then the specified WaveguideCoplanar segment will also use the “base metal addition” layer so that a waveguide trace may continue inside a metal gap region.A notable implementation detail is that every Airbridge (sub)class is done as AirbridgeConnection. This way a waveguide taper is automatically inserted before and after the airbridge so the user does not have to manually add these. Other Node types do not have this feature.
A WaveguideComposite cell has a method
segment_lengths
that returns a list of lengths of each individual regular waveguide segment. Segments are bounded by any element that is not a standard waveguide, such as Airbridge, flip chip, taper or any custom element.For examples see the test_waveguide_composite.lym script.
- classmethod create(layout, library=None, **parameters)[source]
Create cell for this element in layout.
- Parameters:
layout – pya.Layout object where this cell is created
library – LIBRARY_NAME of the calling PCell instance
**parameters – PCell parameters for the element as keyword arguments
- static get_segment_cells(cell)[source]
Get the subcells of a
WaveguideComposite
cell, ordered by node index.The subcells include
WaveguideCoplanar
,WaveguideCoplanarTaper
andMeander
cells for straight, tapered and meandering segments, respectively, and any element cells that were inserted explicitly (element
argument) or implicitly (changinga
,b
orface_id
) at anyNode
.The
node_index
returned with each subcell is an index to thenodes
parameter of theWaveguideComposite
cell. It points to the node that _created_ the subcell, which is the node following a segment for regularWaveguideCoplanar
segments, and the node that specified the element or parameter change otherwise.Note that there may be multiple subcells per node, and some nodes may not have associated subcells (in particular, regular
WaveguideCoplanar
segments are merged when possible). Subscells are returned in the order in which they appear in the waveguide.- Parameters:
cell – A WaveguideComposite cell. Can be a PCell or static cell created from a PCell.
Returns: A list of tuples (node_index: int, subcell: pya.Cell) ordered by node_index.
- static get_segment_lengths(cell)[source]
Retrieves the segment lengths of each waveguide segment in a WaveguideComposite cell.
Waveguide segments are
WaveguideCoplanar
,WaveguideCoplanarTaper
andMeander
subcells. This method returns a list with the same length as thenodes
parameter, where each element is the total length of all waveguides directly preceding and/or defined in that node. For example, for a taper node both the preceding regular waveguide and the taper itself are counted.Note that
WaveguideComposite
merges consecutive waveguide segments if they have no special elements. As a consequence, the corresponding waveguide lengths all accumulate in the next index which has a taper, meander or other element, and the length for nodes that contain onyWaveguideCoplanar
is reported as 0.- Parameters:
cell – A WaveguideComposite cell. Can be a PCell or static cell created from a PCell.
Returns: A list waveguide lengths per node
- static produce_fixed_length_waveguide(chip, route_function, initial_guess=0.0, length=0.0, **waveguide_params)[source]
Produce a waveguide composite with fixed length. route_function should be a single-argument function that returns route, and its argument is an adjustable length in µm. Note that this is not a minimization, but a single-step adjustment that only corrects for the offset in length.
- Parameters:
chip – Chip in which the element is added (self if called within chip code)
route_function – a function lambda x: [Node(f(x))…] where x can be for instance a meander length or a
list (DPoint coordinate (if more than one component is tuned in the Node)
be (the correction length must)
weighted)
initial_guess – float that allows to draw an initial waveguide of a reasonable length
length – target desired length for the final waveguide
waveguide_params – kwargs to be passed to the WaveguideComposite element, such as a, b, term1, term2 etc.
Returns: The waveguide instance, refpoints and the final length
- snap_point(point: DPoint) DPoint [source]
Interface to define snap behavior for GUI editing in derived classes.
- Parameters:
point – DPoint that has been moved in the GUI
- Returns: DPoint representing the snapped location for the input point. Return the point unmodified if no
snapping is required
- kqcircuits.elements.waveguide_composite.produce_fixed_length_bend(element, target_len, point_a, point_a_corner, point_b, point_b_corner, bridges)[source]
Inserts a waveguide bend with the given length to the chip.
- Parameters:
element – The element to which the waveguide is inserted
target_len – Target length of the waveguide
point_a – Endpoint 1 of the waveguide
point_a_corner – Point towards which the waveguide goes from endpoint 1
point_b – Endpoint 2 of the waveguide
point_b_corner – Point towards which the waveguide goes from endpoint 2
bridges – String determining where airbridges are created, “no” | “middle” | “middle and ends”
- Returns:
Instance of the created waveguide
- Raises:
ValueError, if a bend with the given target length and points cannot be created. –
nodes (String) - List of Nodes for the waveguide, default=
(0, 0, 'Airbridge'), (200, 0)
enable_gui_editing (Boolean) - Enable GUI editing of the waveguide path, default=
True
gui_path (Shape) - , default=
(0,0;200,0) w=1 bx=0 ex=0 r=false
gui_path_shadow (Shape) - Hidden path to detect GUI operations, default=
(0,0;200,0) w=1 bx=0 ex=0 r=false
tight_routing (Boolean) - Tight routing for corners, default=
False
ab_to_ab_spacing (Double) - Spacing between consecutive airbridges, default=
500
, unit=μm
ab_to_node_clearance (Double) - Spacing between an airbridge and a node, default=
100
, unit=μm
airbridge_params (String) - Extra params to be passed to the airbridge class, default=
{}
a (Double) - Width of center conductor, default=
10
, unit=μm
b (Double) - Width of gap, default=
6
, unit=μm
n (Int) - Number of points on turns, default=
64
r (Double) - Turn radius, default=
100
, unit=μm
margin (Double) - Margin of the protection layer, default=
5
, unit=μm
face_ids (List) - Chip face IDs list, default=
['1t1', '2b1', '1b1', '2t1']
display_name (String) - Name displayed in GUI (empty for default), default=
protect_opposite_face (Boolean) - This applies only on signal carrying elements that typically include some metal between gaps., default=
False
opposing_face_id_groups (List) - Opposing face ID groups (list of lists), default=
[['1t1', '2b1']]
etch_opposite_face (Boolean) - Etch avoidance shaped gap on the opposite face too, default=
False
etch_opposite_face_margin (Double) - Margin of the opposite face etch shape, default=
5
, unit=μm
ubm_diameter (Double) - Under-bump metalization diameter, default=
40
, unit=μm
bump_diameter (Double) - Bump diameter, default=
25
, unit=μm
connector_type (String) - Connector type, default=
Coax
, choices=['Single', 'GSG', 'Coax']
inter_bump_distance (Double) - Distance between In bumps, default=
100
, unit=μm
output_rotation (Double) - Rotation of output port w.r.t. input port, default=
180
, unit=degrees
connector_a (Double) - Conductor width at the connector area, default=
40
, unit=μm
connector_b (Double) - Gap width at the connector area, default=
40
, unit=μm
round_connector (Boolean) - Use round connector shape, default=
False
n_center_bumps (Int) - Number of center bumps in series, default=
1
a2 (Double) - Non-physical value ‘-1’ means that the default size ‘a’ is used., default=
-1
, unit=μm
b2 (Double) - Non-physical value ‘-1’ means that the default size ‘b’ is used., default=
-1
, unit=μm
term1 (Double) - Termination length start, default=
0
, unit=μm
term2 (Double) - Termination length end, default=
0
, unit=μm
add_metal (Boolean) - Add trace in base metal addition too, default=
False
ground_grid_in_trace (Boolean) - Add ground grid also to the waveguide, default=
False
airbridge_type (String) - Airbridge type, default=
Airbridge Rectangular
, choices=['Airbridge Rectangular', 'Airbridge Multi Face']
taper_length (Double) - Taper length, default=
100
, unit=μm
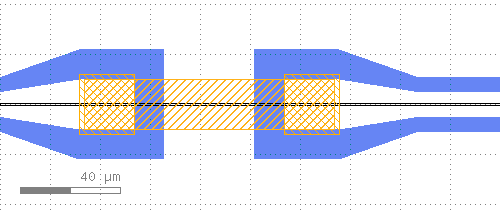